Debugging code is a challenging task. When your code doesn’t work, it can be challenge to find the cause of the problem. You may do things like comment out the code or make a small change to see if that fixes the issue. What do you do when these tricks don’t help you find the bug?
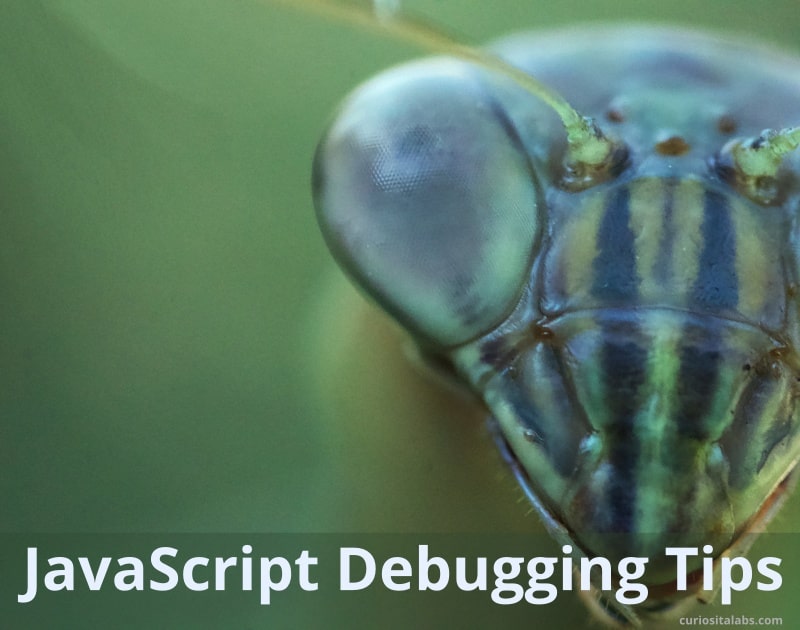
If you are like many JavaScript developers, you start with console.log to find the problems in your code.
When to use console.log()
Console.log() is simple and easy to use debugging tool. You can have it display a message so you know that the code worked. Or you can use variables and expressions to determine if you are getting the right values.
When to use debugger
What do you do if the problem is hard to find? You can use a debugging tool. A debugging tool is a software tool that helps you identify coding errors. You can step through your code line by line to see how it is working. You can stop executing the code at certain points. It can help you to read error messages and handle exceptions.
Your browser has powerful debugging tools include the console.log. Each browser is a bit different on the development tools that it offers. Learn more about the basic functions of your browser’s development tools.
Tips for debugging JavaScript
- Start with the console.log(). You can always switch to using other debugging methods if the console.log doesn’t work for you.
- Read the error message. Error messages may include helpful details. Like the line number where the error occurred, type of error and a brief description.
- Use Breakpoints. Breakpoints allow you to pause your code at a specific line. You can step through your code line by line and see how it is working.
- Add the debugger statement in your code. It acts like a breakpoint in your code. When the code reaches this point, it pauses at debugger. If no debugger functionality is available, your browser ignores this statement.
- Handle Exceptions. Exceptions are errors that when your code is executing. They can occur when you have syntax, logic or runtime errors. Make sure you are handling exceptions. Exceptions can help you to identify and resolve issues in your code.
Debugging like coding takes practice. Use this tips to help you get better at finding issues in your code. If you still can’t find it, take a break. Walking away from the problem sometime help. So can talking to others also helps.